In the second of this two-part blog, learn what “xApps” are and how you can get started with developing them to unleash a new paradigm of innovation in the RAN.
Last month, VMware launched the VMware RIC SDK Partner Program to invite partners to develop applications for the VMware RAN Intelligent Controller (RIC). Through this program, VMware is offering software development kits (SDKs) and a host of other resources to partners to help them develop and operationalize what are known as “rApps” and “xApps” – applications that run with the Non-Real-Time RIC and the Near-Real-Time RIC respectively.
In this blog, we will dive deeper into the following topics:
- What is the Near-Real-Time RIC, and what are xApps?
- How can developers get started with developing xApps using the VMware RIC SDK?
What is the Near-Real-Time RIC, and what are xApps?
Traditionally, the RAN control plane – the set of control algorithms in the RAN processing stack (see figure 1 below) – has been closed and vendor proprietary. These control algorithms, that range from radio resource control (RRC) functions like admission control, mobility control, etc. to medium access control (MAC) functions like PRB allocation, beamforming control, etc., directly impact how efficiently the RAN uses an operator’s valuable spectrum assets and what quality of service (QoS) operators can deliver to their subscribers.
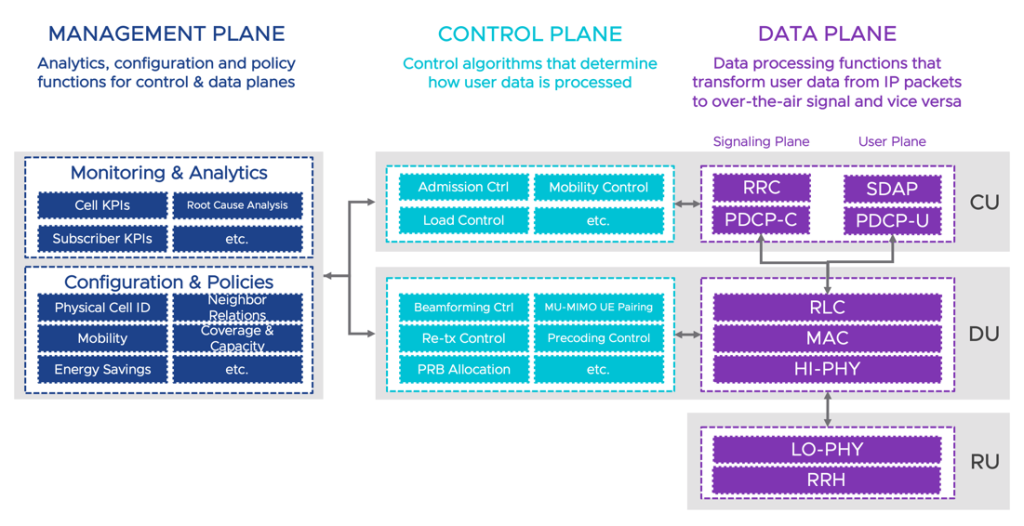
Figure 1: The data, control and management planes of a radio access network (RAN)
With the advent of open RAN, O-RAN Alliance has taken a software-defined approach and made it possible for external developers to program the near-real-time RAN control plane, i.e., the subset of control algorithms that can tolerate control loop delays of 10 milliseconds to 1 second1. The key element in the O-RAN architecture that makes this possible is the Near-Real-Time RAN Intelligent Controller a.k.a. the Near-RT RIC (see figure 2 below).

Figure 2: O-RAN Architecture introduces the Near-RT RIC and the Non-RT RIC to allow external applications to program the control and the management planes of the RAN respectively.
The Near-RT RIC offers a set of services that provides the foundation for a multi-vendor ecosystem to program the control-plane intelligence in the RAN nodes (O-CU, O-DU, etc.). A key service is the E2 termination service which terminates the O-RAN E2 interface from RAN nodes (O-CU, O-DU, etc.) and exposes the ability to program these nodes directly – see figure 3 below for other services in the Near-RT RIC.
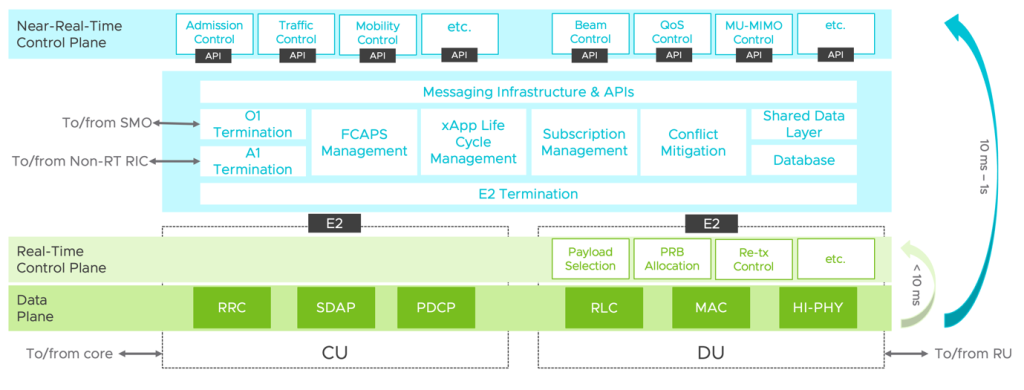
Figure 3: The key services provided by the Near-RT RIC to enable a multi-vendor ecosystem to program the near-real-time control-plane intelligence in the RAN.
The Near-RT RIC services can be extended by what are known as xApps: self-contained applications, which may be provided by third parties, that consume one or more Near-RT RIC services and contain the control-plane intelligence to analyze and/or optimize the RAN.
It is worth highlighting here that the Near-RT RIC and xApps offer a new paradigm of innovation in the RAN. For the first time in the history of commercial RAN, the RAN control plane is open to external developers. Third-party developers – smaller companies, start-ups, universities, research organizations or even individual developers – can now build xApps to program the deeper layers of the RAN, compete to push the frontiers in subscriber QoS, spectral efficiency and other RAN KPIs, and innovate new kinds of monetizable services for operators.
VMware RIC SDK for xApp developers
VMware is on a mission to make the RAN developer friendly and create an arena where developers can thrive, compete, and innovate. To that end, VMware offers xApp developers a software development kit (SDK), which has been designed to simplify the developer effort in all stages (development, build, packaging, integration and testing) of xApp development. Several components of this SDK are programming language specific, and it currently supports C and C++. Based on requests and preferences of our xApp developer partners, we plan to add support for more languages in the future.
As part of the development kit, an xApp developer is provided with the following:
- SDK library: This is a C static archive library which abstracts the messaging mechanism of the xApp with other components in the O-RAN architecture.
- SDK API: Data structures and function calls in C, C++ that serve as an API for the xApp to interact with the SDK library mentioned above. These allow the xApp to send and receive messages to/from other components in the O-RAN architecture.
- xApp development container: A docker container with VMware supported version of Photon OS. It also comes pre-installed with a host of commonly used packages. The xApp developer is recommended to use this container as their development environment.
- Demo xApps: Sample code for several reference xApps that serve as a starting point for xApp developers and demonstrate how to make use of the SDK APIs to build xApps.
- Build tools: Build rules (using Bazel) for building the demo xApp and linking it with the SDK library. The xApp developers have the option of reusing these build rules for their xApps.
- Programmable E2 node: A library (C static archive library) and APIs (C/C++ header files) so xApp developers can build their own simplified E2 nodes (O-CU, O-DU, etc.) for end-to-end testing of their xApps.
- Kubernetes Custom Resource Definition: With VMware Distributed RIC 2.0, xApps are packaged and deployed as a custom resource in the Kubernetes cluster. The custom resource definition (CRD) and one or more sample custom resources (CRs) to be used as a starting point are included.
Hello World xApp
An xApp is packaged as a docker container and described using a Kubernetes Custom Resource (see the demo xApps in SDK for examples of how this is done). This custom resource allows the xApp developer to specify any static configs, CPU and memory requirements as well as the bash command to launch the xApp.
Once launched, the xApp must initialize the SDK. After this, the xApp has access to all the functions exposed by the SDK to interact with other components of the O-RAN architecture. For example, xApps will commonly interact with RAN (E2) nodes, i.e., O-CU and/or O-DU. For facilitating this interaction, the SDK exposes the following functions:
- poll_e2_messages: provides a count of incoming messages from E2 nodes that are pending to be read by the xApp.
- read_e2_message: allows the xApp to read the next pending message from an E2 node. Returns a data structure with the contents of the message as output.
- free_e2_message: allows the xApp to free the memory allocated for the incoming message. This function should be called only after the incoming message has been processed.
- write_e2_message: allows the xApp to send a message to a particular E2 node. Requires a data structure with the contents of the message as an input.
Using these functions, the xApp can interact with the various E2 nodes (O-CU, O-DU) in the RAN. The following flowchart illustrates a typical xApp workflow.
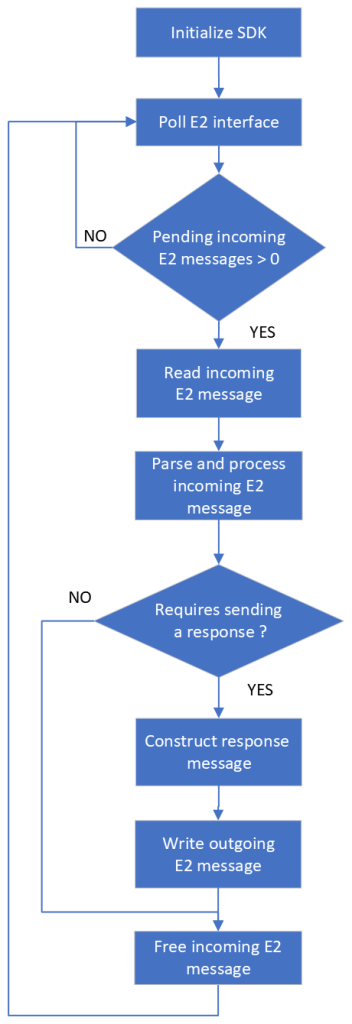
Figure 4: An illustrative xApp workflow
Here are code snippets that illustrate how an xApp developer can implement the above workflow.
- Main function with SDK initialization and an infinite loop where the interfaces are “serviced”.
```
int main ()
{
sdk_init_args_t init_args;
// < Populate init_args >
dric_sdk_api sdk_api(&init_args);
sdk_api.sdk_init(); // Initialize SDK
// < Other logic and initializations >
while (1) {
service_e2_intf(&sdk_api);
// <Service other interfaces >
}
}
```
- “Servicing” of E2 interface involves polling it, and if any incoming messages are pending, they need to be read, “handled” and freed.
```
void
service_e2_intf (dric_sdk_api *sdk_api)
{
s2x_e2_msg_to_xapp_t *rx_msg;
if (sdk_api->poll_e2_messages() > 0) {
rx_msg = sdk_api->read_e2_message();
handle_e2_message(sdk_api, rx_msg);
sdk_api->free_e2_message(rx_msg);
}
}
```
- Finally, “handling” of an incoming message will be use-case specific, but it’s expected to involve parsing the message. If a response is required, it’ll also involve constructing the response and writing it back to the interface.
````
void
handle_e2_message (dric_sdk_api *sdk_api,
s2x_e2_msg_to_xapp_t *rx_msg)
{
// < Parse rx msg >
// < Decide if a response is required >
if (resp_needed) {
s2x_e2_msg_from_xapp_t *resp_msg = construct_response();
sdk_api->write_e2_message(resp_msg, &free_rtn);
}
}
````
Beyond interacting with the E2 nodes in the RAN, the xApp can also use one or more of the following interfaces. The interaction with these interfaces is very similar to the E2 interface interaction described above.
- Management interface: For registering and reporting metrics. This allows the xApp to be monitored and debugged at runtime. Adding support for more comprehensive FCAPS (Fault, Configuration, Accounting, Performance, Security) management is on the roadmap.
- SDL interface: Functions for writing to and reading from the Shared Data Layer.
- A1-related interface (roadmap): Functions for interacting with the Non-RT RIC via the O-RAN A1 interface.
- Utility interface: VMware proprietary interface for additional value-add services.
– One example is registering timers; once a timer is registered, a notification will be sent to the xApp whenever the timer fires – this allows the xApp to perform any periodic tasks that it needs to accomplish its objective.
With the above, an xApp developer can implement a wide variety of intelligent algorithms for the RAN control plane, including advanced analytics applications like precise 5G positioning, innovative optimization algorithms like MU-MIMO pairing and precoding, and new monetizable applications like RAN slice SLA assurance.
For access to the VMware RIC SDK, please reach out to your VMware contact and sign up for the VMware RIC SDK Partner Program. This SDK is an evolving product that will be improved by input from customers and partners. We welcome your questions, comments, and suggestions.
1 – The real-time RAN control plane, i.e., the subset of RAN control algorithms that need sub-10 milliseconds control loops, is not externally programmable in the first generation of the O-RAN defined open RAN architecture. Making the real-time RAN control plane programmable is going to be a challenging but natural evolution of open RAN in a future generation.